This is not a beginner course – if you’ve been coding Python for a week or a couple of months, you probably should keep writing Python for a bit more before File size: 9.72 GBPurchase Python 3: Deep Dive (Part 1 – Functional) courses at here with PRICE $199 $42Python 3: Deep Dive (Part 1 – Functional)What you’ll learnAn in-depth look at variables, memory, namespaces and scopesA deep dive into Python’s memory management and optimizationsIn-depth understanding and advanced usage of Python’s numerical data types (Booleans, Integers, Floats, Decimals, Fractions, Complex Numbers)Advanced Boolean expressions and operatorsAdvanced usage of callables including functions, lambdas and closuresFunctional programming techniques such as map, reduce, filter, and partialsCreate advanced decorators, including parametrized decorators, class decorators, and decorator classesAdvanced decorator applications such as memoization and single dispatch generic functionsUse and understand Python’s complex Module and Package systemIdiomatic Python and best practicesUnderstand Python’s compile-time and run-time and how this affects your codeAvoid common pitfallsGet Python 3: Deep Dive (Part 1 – Functional) downloadCourse contentExpand all 158 lectures44:38:14-Introduction18:55Course OverviewPreview18:08Pre-RequisitesPreview00:37Code Projects and NotebooksPreview00:09-A Quick Refresher – Basics Review02:22:54IntroductionPreview01:43The Python Type Hierarchy05:51Multi-Line Statements and Strings21:49Variable Names11:00Conditionals07:38Functions12:27The While Loop14:25Break, Continue and the Try Statement10:24The For Loop17:20Classes40:17-Variables and Memory03:01:45IntroductionPreview02:54Variables are Memory References08:20Reference Counting14:21Garbage Collection26:38Dynamic vs Static Typing05:28Variable Re-Assignment04:48Object Mutability15:21Function Arguments and Mutability17:28Shared References and Mutability09:36Variable Equality14:22Everything is an Object13:58Python Optimizations: Interning09:13Python Optimizations: String Interning19:10Python Optimizations: Peephole20:08-Numeric Types07:54:31IntroductionPreview02:58Integers: Data Types18:06Integers: Operations24:25Integers: Constructors and Bases – Lecture29:34Integers: Constructors and Bases – Coding20:22Rational Numbers – Lecture14:26Rationals Numbers – Coding12:33Floats: Internal Representations – Lecture19:52Floats: Internal Representations – Coding04:56Floats: Equality Testing – Lecture18:42Floats: Equality Testing – Coding14:40Floats: Coercing to Integers – Lecture09:38Floats: Coercing to Integers – Coding05:03Floats: Rounding – Lecture25:21Floats: Rounding – Coding13:33Decimals – Lecture16:49Decimals – Coding10:26Decimals: Constructors and Contexts – Lecture10:05Decimals: Constructors and Contexts – Coding10:28Decimals: Math Operations – Lecture09:32Decimals: Math Operations – Coding13:30Decimals: Performance Considerations10:29Complex Numbers – Lecture11:28Complex Numbers – Coding14:16Booleans20:59Booleans: Truth Values – LecturePreview09:08Booleans: Truth Values – Coding14:47Booleans: Precedence and Short-Circuiting – Lecture21:10Booleans: Precedence and Short-Circuiting – Coding13:37Booleans: Boolean Operators – LecturePreview18:00Booleans: Boolean Operators – CodingPreview14:45Comparison Operators20:53-Function Parameters04:05:16IntroductionPreview01:05Argument vs Parameter03:43Positional and Keyword Arguments – Lecture13:04Positional and Keyword Arguments – Coding06:21Unpacking Iterables – Lecture13:00Unpacking Iterables – Coding21:09Extended Unpacking – LecturePreview17:50Extended Unpacking – CodingPreview29:04*args – Lecture06:00*args – Coding11:47Keyword Arguments – Lecture09:23Keyword Arguments – Coding14:18**kwargs10:28Putting it all Together – Lecture13:25Putting it all Together – Coding17:25Application: A Simple Function Timer19:08Parameter Defaults – Beware!!18:44Parameter Defaults – Beware Again!!19:22-First-Class Functions05:18:53IntroductionPreview04:05Docstrings and Annotations – Lecture15:58Docstrings and Annotations – Coding15:02Lambda Expressions – LecturePreview12:09Lambda Expressions – Coding14:59Lambdas and Sorting15:56Challenge – Randomize an Iterable using Sorted!!02:55Function Introspection – Lecture19:30Function Introspection – Coding28:35Callables14:46Map, Filter, Zip and List Comprehensions – Lecture21:42Map, Filter, Zip and List Comprehensions – Coding21:14Reducing Functions – Lecture25:51Reducing Functions – Coding21:10Partial Functions – Lecture11:12Partial Functions – Coding25:32The operator Module – Lecture15:34The operator Module – Coding32:43-Scopes, Closures and Decorators08:34:18IntroductionPreview01:31Global and Local Scopes – Lecture34:54Global and Local Scopes – Coding15:40Nonlocal Scopes – Lecture22:17Nonlocal Scopes – Coding14:36Closures – Lecture38:35Closures – Coding32:04Closure Applications – Part 115:37Closure Applications – Part 218:40Decorators (Part 1) – Lecture21:06Decorators (Part 1) – Coding20:58Decorator Application (Timer)35:16Decorator Application (Logger, Stacked Decorators)23:46Decorator Application (Memoization)29:14Decorators (Part 2) – Lecture11:44Decorators (Part 2) – Coding25:57Decorator Application (Decorator Class)09:40Decorator Application (Decorating Classes)48:23Decorator Application (Dispatching) – Part 131:45Decorator Application (Dispatching) – Part 235:45Decorator Application (Dispatching) – Part 326:50-Tuples as Data Structures and Named Tuples03:31:18IntroductionPreview03:18Tuples as Data Structures – Lecture19:01Tuples as Data Structures – Coding25:24Named Tuples – Lecture27:48Named Tuples – Coding35:13Named Tuples – Modifying and Extending – Lecture14:25Named Tuples – Modifying and Extending – Coding21:46Named Tuples – DocStrings and Default Values – Lecture13:30Named Tuples – DocStrings and Default Values – Coding15:46Named Tuples – Application – Returning Multiple Values06:22Named Tuples – Application – Alternative to Dictionaries28:45-Modules, Packages and Namespaces05:43:11IntroductionPreview03:01What is a Module?24:30How does Python Import Modules?49:32Imports and importlib27:39Import Variants and Misconceptions – Lecture13:59Import Variants and Misconceptions – Coding27:03Reloading Modules18:29Using __main__27:01Modules Recap13:02What are Packages? – Lecture20:24What are Packages ? – Coding27:11Why Packages?13:07Structuring Packages – Part 136:41Structuring Packages – Part 227:27Namespace Packages10:37Importing from Zip Archives03:28-Extras03:47:12IntroductionPreview03:40Additional ResourcesPreview12:53Python 3.6 HighlightsPreview07:49Python 3.6 – Dictionary OrderingPreview19:45Python 3.6 – Preserved Order of kwargs and Named Tuple Application05:32Python 3.6 – Underscores in Numeric Literals03:38Python 3.6 – f-Strings09:19Random: Seeds17:26Random Choices26:07Random Samples07:02Timing code using *timeit*16:17Don’t Use *args and **kwargs Names Blindly07:35Command Line Arguments01:00:07Sentinel Values for Parameter Defaults11:02Simulating a simple switch in Python19:00RequirementsBasic introductory knowledge of Python programming (variables, conditional statements, loops, functions, lists, tuples, dictionaries, classes).You will need Python 3.6 or above, and a development environment of your choice (command line, PyCharm, Jupyter, etc.)Hello!This is Part 1 of a series of courses intended to dive into the inner mechanics and more complicated aspects of Python 3.This is not a beginner course – if you’ve been coding Python for a week or a couple of months, you probably should keep writing Python for a bit more before tackling this series.On the other hand, if you’re now starting to ask yourself questions like:I wonder how this works?is there another way of doing this?what’s a closure? is that the same as a lambda?I know how to use a decorator someone else wrote, but how does it work? Can I write my own?why isn’t this boolean expression returning a boolean value?what does an import actually do, and why am I getting side effects?and similar types of question…then this course is for you.Please make sure you review the pre-requisites for this course – although I give a brief refresh of basic concepts at the beginning of the course, those are concepts you should already be very comfortable with as you being this course.In this course series, I will give you a much more fundamental and deeper understanding of the Python language and the standard library.Python is called a “batteries-included” language for good reason – there is a ton of functionality in base Python that remains to be explored and studied.So this course is not about explaining my favorite 3rd party libraries – it’s about Python, as a language, and the standard library.In particular this course is based on the canonical CPython. You will also need Jupyter Notebooks to view the downloadable fully-annotated Python notebooks.It’s about helping you explore Python and answer questions you are asking yourself as you develop more and more with the language.In Python 3: Deep Dive (Part 1) we will take a much closer look at:Variables – in particular that they are just symbols pointing to objects in memoryNamespaces and scopePython’s numeric typesPython boolean type – there’s more to a simple or statement than you might think!Run-time vs compile-time and how that affects function defaults, decorators, importing modules, etcFunctions in general (including lambdas)Functional programming techniques (such as map, reduce, filter, zip, etc)ClosuresDecoratorsImports, modules and packagesTuples as data structuresNamed tuplesTo get the most out of this course, you should be prepared to pause the coding videos, and attempt to write code before I do! Sit back during the concept videos, but lean in for the code videos!And after you have seen a code video, pause the course, and try things out yourself – explore, experiment, play with code, and see how things work (or don’t work! – that’s also a great way to learn!)Who this course is for:Anyone with a basic understanding of Python that wants to take it to the next level and get a really deep understanding of the Python language and its data structures.Anyone preparing for an in-depth Python technical interview.Get Python 3: Deep Dive (Part 1 – Functional) downloadPurchase Python 3: Deep Dive (Part 1 – Functional) courses at here with PRICE $199 $42
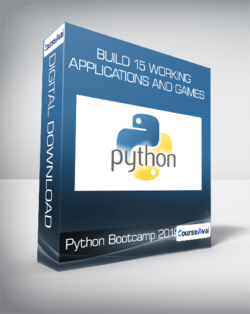
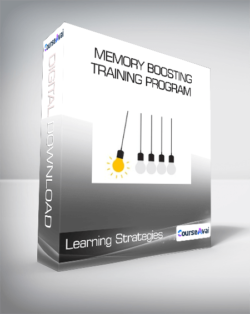
Python 3: Deep Dive (Part 1 – Functional)
₹6,972.00